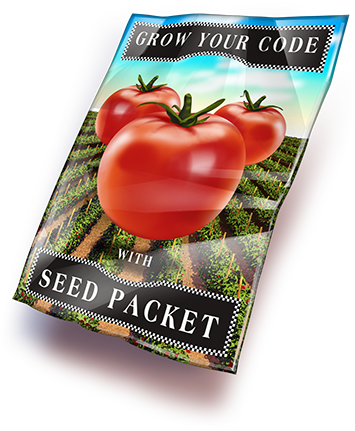
SeedPacket
Lightning-Fast LINQ Data Generation for .NET 8/9
SeedPacket quickly seeds data in .NET for mockups, RAD, testing, prototyping, and database seeding. Written in C#, it features an easy-to-use, customizable rules engine that supports external data sources like XML, JSON, or strings.
How It Works
SeedPacket extends IEnumerable with a .seed() method, similar to LINQ, to populate lists with fully formed elements. The rules engine uses data types, interfaces, and property names to generate relevant data—emails for email fields, phone numbers for phone fields, and so on.
By default, it applies 30+ common rules and falls back to generic ones if needed. Custom rules are easy to create and modify, with examples provided, including integration with external data sources. Generated data can be static across requests or randomized each time.
Simple Example
Creating seed data is as simple as importing SeedPacket from Nuget, adding the SeedPacket.Extensions namespace, and calling .Seed() on an existing an IEnumerable such as List, etc.
The adjacent User Data Example is generated using the second example below. Adjust the Rows and Seed values in the Try it out! box to update in real time.
// Example 'User' Class
public class User
{
public int UserId { get; set; }
public string FirstName { get; set; }
public string LastName { get; set; }
public string Email { get; set; }
public DateTime Created { get; set; }
}
// Creates 10 rows (default)
var users = new List<User>().Seed();
// Create 20 rows (see the 'User Data Example')
var users = new List<User>().Seed(20);
// Create rows starting with 100 to 200
var users = new List<User>().Seed(100, 200);
Try it out!
Update these values to see the Users Example update in real time.
Customization is simple—SeedPacket’s rules engine can modify generated data using custom rules. The base data comes from embedded source code but can be overridden with an external XML/JSON file or string.
By default, well-formed usernames and emails are generated based on property types and names. For example, if a property is a string containing "email" or "username," it generates appropriate data, even constructing usernames from first and last names. Other types, like DateTime (e.g., "Created") or int (e.g., "Id"), are also handled. Rules prioritize matches by data type first, then property name.
By default, the data remains consistent between each call as it determined by a base RandomNumber that is seeded with a static value. All other random values are derived from this number for each row of data, creating a "random tree". Passing in a non-static random value at the root of the tree will cause all values to be different each time.
The real power lies in custom rules, which we'll explore next—first, let’s look at a more advanced example.
UserId | FirstName | LastName | |
---|---|---|---|
1 | Theresa | Martinez | tmartinez@nakatomiindustries.com |
2 | Peter | Edwards | pedwards@tyrell.com |
3 | Summer | Gonzalez | sgonzalez@globaxco.com |
4 | Jacqueline | Hill | jhill@starkco.com |
5 | Crystal | Allen | callen@primosystems.com |
6 | Jesse | Collins | jcollins@primocorp.com |
7 | Patricia | Hernandez | phernandez@capitalco.com |
8 | Theresa | Moore | tmoore@multinationalcorporation.com |
9 | Crystal | Martinez | cmartinez@multinationalco.com |
10 | Sarah | Adams | sadams@parallaxco.com |
11 | Theresa | Walker | twalker@nakatomico.com |
12 | Omar | Lewis | olewis@umbrellainc.com |
13 | Janice | Carter | jcarter@compudataco.com |
14 | Denise | Wilson | dwilson@megaindustries.com |
15 | John | Anderson | janderson@nextind.com |
16 | Dylan | Davis | ddavis@oscorp.com |
17 | Phillip | Lewis | plewis@wonkacorp.com |
18 | Susan | Gonzalez | sgonzalez@globaxco.com |
19 | Nicole | Wright | nwright@holdingsco.com |
20 | William | Rodriguez | wrodriguez@sunrise.com |
Installing SeedPacket
The source code is available on: GitHub
Install using the NuGet Package Manager in Visual Studio:
PM> Install-Package SeedPacket
For more details: NuGet - SeedPacket
Advanced Example
For more advanced situations, you can pass in a generator class that contains a customizable rules engine. By default, the "Advanced" ruleset is used which contains about thirty common rules and the data is loaded from an internal resource. In the example below a "MultiGenerator" is injected into the Seed method.
var generator = new MultiGenerator("~/SourceFiles/xmlSeedSourcePlus.xml")
{
Rules =
{
new Rule(typeof(string), "ItemName", g => Funcs.GetNextElement(g, "ProductName"), "ItemName"),
new Rule(typeof(int), "SelectedProduct", g => g.RowRandom.Next(0, 11), "Random product id"),
new Rule(typeof(string), "Ceo", g => Funcs.GetNextElement(g, "FirstName") + " " + func.NextElement(g, "LastName"), "Random CEO Name"),
new Rule(typeof(string),"Description%", g => Funcs.GetRandomElement(g, "Description"), "Description", "Gets Description from custom XML file" ),
new Rule(typeof(List<Item>), "", g => Funcs.GetCacheItemsNext<Item>(g, g.Cache.Items, 10, 10, false), "ItemList"),
},
BaseDateTime = DateTime.Today,
BaseRandom = new Random(1234567)
};
generator.Cache.Items = new List<Item>().Seed(1, 10, generator);
var examples = new List<Example>().Seed(100, 115, generator);
- The first rule applies to the "ItemName" field uses a built-in seedpacket function (Func) that gets the next element from the datasource list "ProductName". It actually overrides a rule that gets random (fake) products and replaces it with one that gets the next item in the sequence. This insures the values will all be unique, if the number of items requested is less than in the source.
- The second rule, randomly picks a selected value for the dropdown from 0 to 10 (the C# Random() method picks values less than the max value).
- The third rule, randomly picks a first and last name for any string fields matching "CEO"
- The fourth rule, matches any string starting with "Description" and gets a random value from the datasoure called "Description". This is not in the default lists that are "built-in" in SeedPacket. In the constructor for the MultiGenerator, you will see that we are now passing in a custom xml file that has all the source data for our lists. Now we have complete control of our source data and Funcs.RandomElement() can pull from any named element.
- The fifth custom rule is the most interesting and makes sure that any List<Item> fields are filled from a generated list that has been saved in the generator's Cache. We can populate this generated list from another .Seed call and this allows us to have complex, hierarchical data when needed. Note how we can initially declare the rules, but then need to make sure we fill the cached data before the rules are actually run.
- Notice how we set BaseDateTime as the starting point for all generated DateTime values. Similarly, BaseRandom serves as the root of the "Random Tree," influencing all other random values like RowRandom.
Try it out!
Update these values to see the Companys Example update in real time.
Id | Company | City | State | CEO | NetWorth | Products | Description | CompanyGuid |
---|---|---|---|---|---|---|---|---|
100 | Spacely Co | York | AZ | Collins | $365.33M | Square, man | 26808641-6dad-d0e7-aae8-ce5c9974c7f6 | |
101 | Sunrise Co | Peachtree | PA | John Smith | $432.91M | Smelly but good | 1c486fb6-df51-7f00-7fca-9b1a36474f14 | |
102 | Umbrella Ind | Sierra | CO | Patricia Johnson | $451.34M | Awful | 915bac43-c847-da17-0cd3-c5279b12c49c | |
103 | Oscorp | Winterspring | KY | Michael Williams | $601.67M | Rich! | 5a030f6c-7cbe-781a-9bc9-5ef75a6a2307 | |
104 | Acme | Stone Bridge | MS | Susan Jones | $830.81M | Who knows | cf046fd8-e63b-1f0c-1f51-5b26eab1ce49 | |
105 | Mega | Brighton | AL | William Brown | $303.03M | Bumpy | 70a97f90-ee1e-9e2b-d37b-7d37e9882764 | |
106 | Oscorp Co | Franklin | OK | Mary Davis | $140.55M | Angular | f517eb9b-b3da-09a4-4376-9615a1d34423 | |
107 | City Corporation | Lincoln | ND | Robert Miller | $42.36M | Complicated | 356968d7-716d-ee35-5d48-5621266c44d0 | |
108 | CyberGlobe LLC | Oakleaf | TX | Sarah Wilson | $735.37M | Angular | f1686106-c758-522b-0b1b-e08e1b4796f6 | |
109 | Virtucon Co | Washington | FL | Peter Moore | $873.04M | Transparent | 18de332b-2d5d-0593-13ff-f347306ce729 |